Part 3: Chart Overlay – Pinescript Tutorial Basics
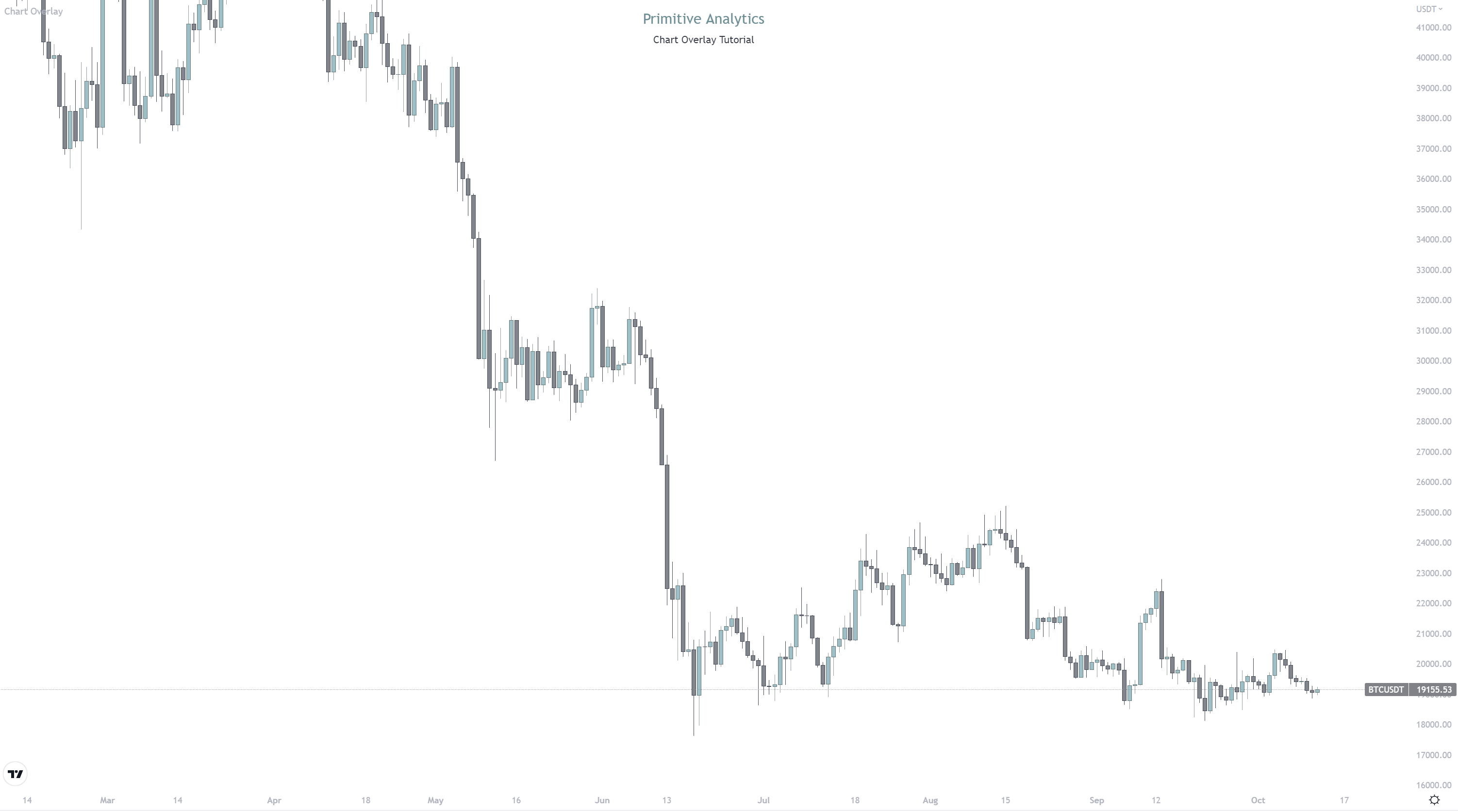
Before we get started in you haven’t read my short introduction see this post here: About Me
Previous Posts in Series:
- Part 1: Chart Overlay – Pinescript Tutorial Basics
- Part 2: Chart Overlay – Pinescript Tutorial Basics
Pinescript version 5
Useful links:
Goal
Add input functions to a simple chart overlay text indicator using Pinescript.
Tutorial Introduction
In this final installment we will complete this pinescript tutorial of a chart overlay indicator for Trading View using the following functions:
The process if fairly straight forward.
- Maintain the general sub heading input from the previous script
- Add text inputs for each day of the week
- Add a toggle so the user can switch between the text changing by the day of the week and one that is constant for all days
- Logic in the code to detect day of the week and use appropriate text
Here is where we left off in Part 2: Chart Overlay – Pinescript Tutorial Basics
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
//@version=5
indicator("Chart Overlay", overlay = true)
//inputs
//text inputs
headingString = input.string("Input text here")
subHeadingString = input.string("Input text here")
//colour inputs
headingColour = input.color(color.black, title="Heading Colour")
subHeadingColour = input.color(color.black, title="Sub-Heading Colour")
//initialize table element
var overlayTable = table.new(position = position.top_center, columns = 1, rows = 2)
if barstate.islast
table.cell(table_id = overlayTable, column = 0, row = 0, text = headingString, text_color = headingColour, text_size = size.large)
table.cell(table_id = overlayTable, column = 0, row = 1, text = subHeadingString, text_color = subHeadingColour, text_size = size.normal)
Day of the Week Text Elements
Let us begin by adding in the text elements for each day of the week. For this indicator we will keep the heading the same and only change the sub heading based on the day of the week. For the input.string() function the arguments are as follows:
input.string(defval, title, options, tooltip, inline, group, confirm) → input string
We will add titles to each of the inputs this time around to make the Settings UI a bit cleaner. Create an input variable for each day of the week sub heading.
//text inputs days of the week
//Monday
subHeadingStringMon = input.string("Input Monday text here", "Monday Text")
//Tuesday
subHeadingStringTues = input.string("Input Tuesday text here", "Tuesday Text")
//Wednesday
subHeadingStringWed = input.string("Input Wednesday text here", "Wednesday Text")
//Thursday
subHeadingStringThur = input.string("Input Thursday text here", "Thursday Text")
//Friday
subHeadingStringFri = input.string("Input Friday text here", "Friday Text")
//Saturday
subHeadingStringSat = input.string("Input Saturday text here", "Saturday Text")
//Sunday
subHeadingStringSun = input.string("Input Sunday text here", "Sunday Text")
Toggle for Day of the Week Text Elements
Next we’ll add a toggle so the user can select to either use the text for each day of the week or one sub heading for the whole week. For this we will use the input.bool() function. default to the value to false or off – meaning the days of the week text will not be shown, only the same text everyday.
//general or day of the week toggle
toggleDayOfWeek = input.bool(false, "Toggle Days of the Week")
Final step is to add in the logic and clean up the code. The logic is a simple if statement. if the toggle is false use the general sub heading string, else use one of the day of the week strings. To detect the date we will use the built-in function dayofweek() and pass the argument timenow, which is a built-in variable to pull the current time. The dayofweek() function returns an integer but we can also use the built-in named constants dayofweek.monday etc to make the code more readable.
//logic for Day of the Week
if (toggleDayOfWeek == false)
subHeadingString := subHeadingString
else if dayofweek(timenow)==dayofweek.monday
subHeadingString := subHeadingStringMon
else if dayofweek(timenow)==dayofweek.tuesday
subHeadingString := subHeadingStringTues
else if dayofweek(timenow)==dayofweek.wednesday
subHeadingString := subHeadingStringWed
else if dayofweek(timenow)==dayofweek.thursday
subHeadingString := subHeadingStringThur
else if dayofweek(timenow)==dayofweek.friday
subHeadingString := subHeadingStringFri
else if dayofweek(timenow)==dayofweek.saturday
subHeadingString := subHeadingStringSat
else if dayofweek(timenow)==dayofweek.sunday
subHeadingString := subHeadingStringSun
Final Steps: Code Cleanup
In cleaning up the code we’ll add some section breaks in the settings UI. To do this we will use the group argument in each of the inputs as well as create variables for the groups so that we can rename them in a single location if needed.
var GRP1 = "Heading"
var GRP2 = "Days of the Week Text"
Add the group argument to each input.
//inputs
//text inputs Heading
headingString = input.string("Input text here", "Heading", group = GRP1)
//text inputs Sub-headings
//General sub
subHeadingString = input.string("Input text here", "General Sub-Heading Text", group = GRP1)
//general or day of the week toggle
toggleDayOfWeek = input.bool(false, "Toggle Days of the Week", group = GRP2)
//text inputs days of the week
//Monday
subHeadingStringMon = input.string("Input Monday text here", "Monday Text", group = GRP2)
//Tuesday
subHeadingStringTues = input.string("Input Tuesday text here", "Tuesday Text", group = GRP2)
//Wednesday
subHeadingStringWed = input.string("Input Wednesday text here", "Wednesday Text", group = GRP2)
//Thursday
subHeadingStringThur = input.string("Input Thursday text here", "Thursday Text", group = GRP2)
//Friday
subHeadingStringFri = input.string("Input Friday text here", "Friday Text", group = GRP2)
//Saturday
subHeadingStringSat = input.string("Input Saturday text here", "Saturday Text", group = GRP2)
//Sunday
subHeadingStringSun = input.string("Input Sunday text here", "Sunday Text", group = GRP2)
Putting this all together you should have the complete script where the sub heading can dynamically change for each day of the week or not if desired. With a UI that looks like the following:


Thanks for following along with this pinescript tutorial – I hope to complete more of these as I grow my own knowledge of Pinescript.
Complete Script
Complete script is below:
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
//@version=5
indicator("Chart Overlay", overlay = true)
var GRP1 = "Heading"
var GRP2 = "Days of the Week Text"
//inputs
//text inputs Heading
headingString = input.string("Input text here", "Heading", group = GRP1)
//text inputs Sub-headings
//General sub
subHeadingString = input.string("Input text here", "General Sub-Heading Text", group = GRP1)
//general or day of the week toggle
toggleDayOfWeek = input.bool(false, "Toggle Days of the Week", group = GRP2)
//text inputs days of the week
//Monday
subHeadingStringMon = input.string("Input Monday text here", "Monday Text", group = GRP2)
//Tuesday
subHeadingStringTues = input.string("Input Tuesday text here", "Tuesday Text", group = GRP2)
//Wednesday
subHeadingStringWed = input.string("Input Wednesday text here", "Wednesday Text", group = GRP2)
//Thursday
subHeadingStringThur = input.string("Input Thursday text here", "Thursday Text", group = GRP2)
//Friday
subHeadingStringFri = input.string("Input Friday text here", "Friday Text", group = GRP2)
//Saturday
subHeadingStringSat = input.string("Input Saturday text here", "Saturday Text", group = GRP2)
//Sunday
subHeadingStringSun = input.string("Input Sunday text here", "Sunday Text", group = GRP2)
//colour inputs
headingColour = input.color(color.black, title="Heading Colour")
subHeadingColour = input.color(color.black, title="Sub-Heading Colour")
//initialize table element
var overlayTable = table.new(position = position.top_center, columns = 1, rows = 2)
//logic for Day of the Week
if (toggleDayOfWeek == false)
subHeadingString := subHeadingString
else if dayofweek(timenow)==dayofweek.monday
subHeadingString := subHeadingStringMon
else if dayofweek(timenow)==dayofweek.tuesday
subHeadingString := subHeadingStringTues
else if dayofweek(timenow)==dayofweek.wednesday
subHeadingString := subHeadingStringWed
else if dayofweek(timenow)==dayofweek.thursday
subHeadingString := subHeadingStringThur
else if dayofweek(timenow)==dayofweek.friday
subHeadingString := subHeadingStringFri
else if dayofweek(timenow)==dayofweek.saturday
subHeadingString := subHeadingStringSat
else if dayofweek(timenow)==dayofweek.sunday
subHeadingString := subHeadingStringSun
if barstate.islast
table.cell(table_id = overlayTable, column = 0, row = 0, text = headingString, text_color = headingColour, text_size = size.large)
table.cell(table_id = overlayTable, column = 0, row = 1, text = subHeadingString, text_color = subHeadingColour, text_size = size.normal)